Mastering Vision Transformers: Implementation with PyTorch
Written on
Chapter 1: Introduction to Vision Transformers
The groundbreaking paper "Attention is All You Need" transformed the landscape of Natural Language Processing (NLP), leading to the widespread adoption of Transformer-based models for various NLP tasks. It was inevitable that this attention-driven approach would be explored in Computer Vision as well, prompting researchers to achieve state-of-the-art results using Transformer architectures.
Even though convolutional neural networks (CNNs) continue to dominate image classification, the research titled "An Image is Worth 16x16 Words: Transformers for Image Recognition at Scale" reveals that we can effectively utilize pure Transformers on sequences of image patches, challenging the traditional reliance on CNNs.
How does this work?
At a high level, the image is divided into patches, and a sequence of linear embeddings from these patches is fed into a Transformer model. These image patches function similarly to tokens in NLP.
However, unlike CNNs, which possess innate biases such as locality, Transformers do not generalize as effectively when trained on limited datasets. Nonetheless, when trained on substantial datasets, they can achieve or surpass state-of-the-art performance across numerous image recognition benchmarks.
Before we delve into the implementation, I recommend reviewing "The Illustrated Transformer" if you are unfamiliar with Transformer architectures. For a practical example, you can check the project repository linked below.
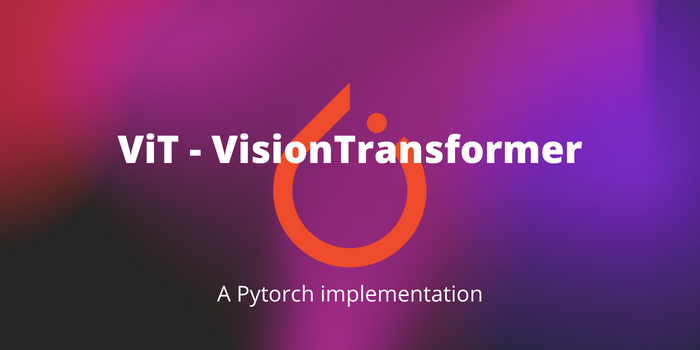
Chapter 2: Implementing Vision Transformer in PyTorch
In this chapter, we will walk through the implementation of the Vision Transformer (ViT) in PyTorch, step by step.
First, we need to import the necessary libraries and prepare an image for testing:
import torch
from einops import rearrange
# Additional imports...
Next, we perform some preprocessing on the image, resulting in a tensor of shape torch.Size([1,3,224,224]).
Subsection 2.1: Breaking Down the Image into Patches
To process the image, we will split it into smaller patches. Here's how we can achieve this using the einops library:
patch_size = 16
patches = rearrange(x, 'b c (h s1) (w s2) -> b (h w) (s1 s2 c)', s1=patch_size, s2=patch_size)
Subsection 2.2: Projecting Patches with Embeddings
The next step involves projecting these patches. While a standard linear layer could suffice, the paper suggests using a convolutional layer for better performance. We will encapsulate this functionality within a PatchEmbedding class.
To verify our implementation, we can call:
PatchEmbedding()(x).shape # Should return torch.Size([1, 196, 768])
Subsection 2.3: Incorporating CLS Token and Position Embedding
Similar to BERT's class token, we prepend a learnable embedding to the sequence of embedded patches, and add position embeddings to retain spatial information.
Subsection 2.4: Understanding the Transformer Encoder
The Transformer encoder consists of alternating layers of multi-headed self-attention and MLP blocks, with layer normalization and residual connections applied accordingly.
# Example of attention mechanism
We will create a wrapper for the residual addition, ensuring smooth integration between the output of the attention block and the fully connected layer.
Subsection 2.5: Finalizing the Vision Transformer Architecture
By assembling all components developed so far, we will construct the complete Vision Transformer model. We can utilize torchsummary to check the model summary:
print(summary(ViT(), (3,224,224), device='cpu'))
Chapter 3: Practical Applications and Conclusion
In this section, we will summarize our findings and compare our results with current state-of-the-art models. If you are interested in seeing a practical implementation, the following video resources will be invaluable.
This video, "ViT (Vision Transformer) Implementation from Scratch with PyTorch!", provides a hands-on approach to building Vision Transformers.
In this video, "Vision Transformer in PyTorch", you will find further insights and applications of Vision Transformers.
By following this guide, we have successfully learned how to implement the Vision Transformer in PyTorch and explored its potential in the realm of computer vision.