How to Retrieve User's Current Location Using JavaScript
Written on
Chapter 1: Introduction to Geolocation in JavaScript
In many development scenarios, it becomes necessary to accurately pinpoint a user's location using JavaScript. This feature is crucial in situations where user input is impractical, and precise location information is desired.
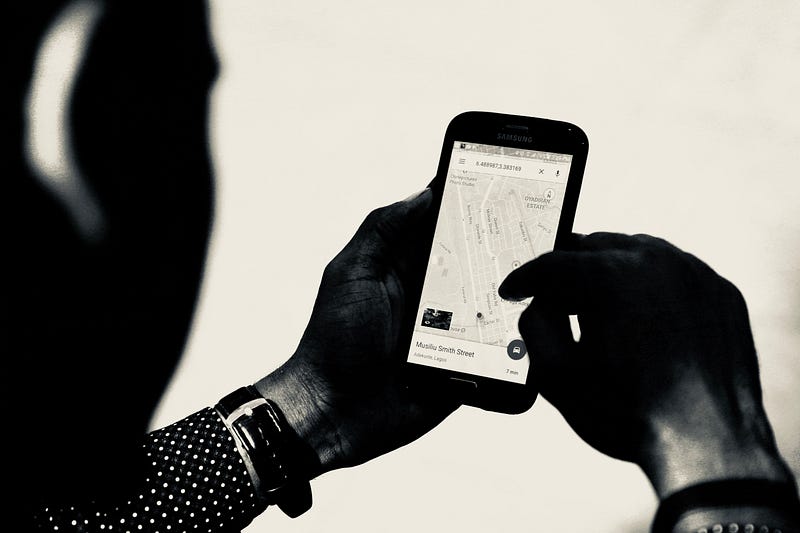
Photo by Desola Lanre-Ologun on Unsplash
Modern web browsers prioritize user privacy and do not permit location tracking without explicit consent. When a website attempts to access location data, users are presented with a prompt to allow or deny access.
Section 1.1: Understanding the Geolocation API
The Geolocation API provides a mechanism for users to share their exact location when permission is granted. To utilize this API, we invoke navigator.geolocation, which intelligently selects the best method to ascertain the device's current position.
For location retrieval, two primary methods are available: geolocation.getCurrentPosition() retrieves a one-time location snapshot, while geolocation.watchPosition() continuously monitors the user's location and updates it as needed.
Subsection 1.1.1: Getting the Current Device Position
// Geolocation options
const geolocationOptions = {
enableHighAccuracy: true, // Requests the most accurate location available.
timeout: 5000, // Sets a timeout for location retrieval.
maximumAge: 0, // Ensures that a fresh position is requested each time.
};
// Function to handle successful geolocation retrieval
function handleGeolocationSuccess(position) {
const coordinates = position.coords;
// Display latitude, longitude, and accuracy
console.log("Your current position is:");
console.log(Latitude: ${coordinates.latitude});
console.log(Longitude: ${coordinates.longitude});
console.log(Accuracy: ${coordinates.accuracy} meters.);
}
// Function to handle geolocation error
function handleGeolocationError(error) {
console.warn(ERROR(${error.code}): ${error.message});
}
// Reference to hold the current location
const currentLocation = ref(null);
// Get the current location using geolocation
navigator.geolocation.getCurrentPosition(handleGeolocationSuccess, handleGeolocationError, geolocationOptions);
The navigator.geolocation.getCurrentPosition() method accepts up to three parameters:
- Success Callback: This function is executed with a GeolocationPosition object upon successful location retrieval, providing access to the necessary data.
- Error Callback: This optional function runs when the location retrieval fails, providing information about the error encountered.
- Options Object: This optional parameter allows the specification of certain options for the position data retrieval.
By obtaining the user's latitude and longitude, we can accurately determine their location on the globe. This information can then be utilized in various APIs to convert the coordinates into a more recognizable format.
However, it is vital to remember that the user must grant permission for location access, as this is a fundamental requirement for safeguarding their privacy.
The Geolocation API is widely compatible with modern web browsers.
Chapter 2: Practical Implementation and Examples
In this first video, "Get users location with Javascript geolocation," viewers can learn how to effectively implement geolocation features in their applications.
The second video, "How to Get a User's Current Location from the Browser with the Geolocation API - JavaScript," offers further insights into leveraging the Geolocation API for user location retrieval.
Conclusion
In summary, we have explored the methods to obtain the real-time location of a device, which directly reflects the user's current position.
Your feedback and support in sharing this information would be greatly appreciated. If you have any inquiries or suggestions for additional content, please feel free to reach out at [email protected] or follow me on Twitter @amhjohnphilip.
More Reads
- How To Pass Custom Headers When Making HTTP Requests in Vue.js
- The Last TypeScript Validation Library You’ll Ever Need!
For more content, visit PlainEnglish.io. Sign up for our free weekly newsletter and follow us on Twitter, LinkedIn, YouTube, and Discord.