Mastering Multi-Domain Routing with Django-Hosts: A Comprehensive Guide
Written on
Chapter 1: Introduction to Django and Multi-Domain Routing
Django is a robust Python web framework that excels in rapid web application development. However, its native URL dispatcher can struggle when faced with the complexities of managing multiple subdomains or distinct domains. This is where the Django-Hosts extension becomes invaluable.
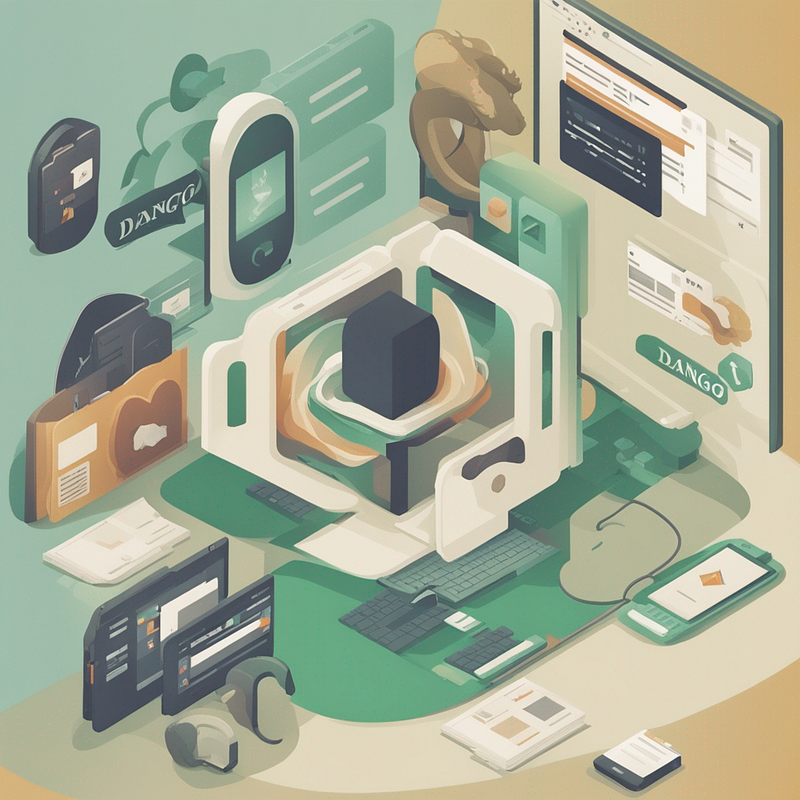
What is Django-Hosts?
Django-Hosts is an open-source tool that enhances Django's functionality by enabling multi-domain and multi-subdomain routing. It allows developers to host various sections of their application across different subdomains or domains. For instance, a blog could be hosted at blog.example.com while an online store could reside at shop.example.com, all under one Django project.
For further details, visit the project on GitHub:
Installation and Setup
Installing Django-Hosts is a simple process. You can do this via pip:
pip install django-hosts
Once installed, update your settings.py file by adding 'django_hosts.middleware.HostsRequestMiddleware' at the beginning and 'django_hosts.middleware.HostsResponseMiddleware' at the end of your MIDDLEWARE list. Additionally, include 'django_hosts' in your INSTALLED_APPS:
MIDDLEWARE = [
'django_hosts.middleware.HostsRequestMiddleware',
# ...
'django_hosts.middleware.HostsResponseMiddleware',
]
INSTALLED_APPS = [
# ...
'django_hosts',
# ...
]
Defining Hosts
To set up hosts, create a file named hosts.py in your project directory. In this file, you can specify your host patterns:
from django_hosts import patterns, host
host_patterns = patterns(
'',
host(r'blog', 'blog.urls', name='blog'),
host(r'shop', 'shop.urls', name='shop'),
)
Specify the ROOT_HOSTCONF setting to point to the module containing your host patterns:
ROOT_HOSTCONF = 'mysite.hosts'
You can also define a DEFAULT_HOST to serve as the fallback pattern if no other matches are found:
DEFAULT_HOST='blog'
Routing and Reverse URL Matching
Routing to various subdomains is straightforward. You can define URL patterns in your usual urls.py files and utilize Django's reverse function to generate URLs for different hosts.
from django_hosts.resolvers import reverse
def some_view(request):
blog_url = reverse('blog_post', host='blog')
# Generates http://blog.example.com/
Using the 'host_url' Template Tag
The host_url template tag lets you generate URLs for specific hosts, adding an extra layer of functionality to Django's built-in url tag.
{% load hosts %}
<!-- Generate URL for the 'blog' subdomain -->
<a href="{% host_url 'blog_post' host 'blog' %}">Blog Post</a>
<!-- Generate URL for the 'shop' subdomain with arguments -->
<a href="{% host_url 'product_detail' host 'shop' product.id %}">Product Detail</a>
If the host parameter isn't specified, the template tag will default to the host defined in the DEFAULT_HOST setting.
Advantages and Use-Cases
- Separation of Concerns: Isolate different application parts into their respective subdomains for better organization.
- SEO Benefits: Subdomains are treated as distinct sites by search engines, which can improve your SEO strategy.
- Ease of Maintenance: Manage and deploy different application parts independently, simplifying overall maintenance.
Chapter 2: Practical Examples and Implementation
To see Django-Hosts in action, check out the following videos:
In this video, the host explains how to utilize Django-hosts for developing multi-tenant websites, showcasing practical examples and usage scenarios.
This video covers the specifics of using Django Hosts for effective subdomain management, providing in-depth insights into its implementation.
Conclusion
Django-Hosts is a valuable extension for enhancing Django's routing capabilities, facilitating multi-domain and multi-subdomain applications. It offers a systematic approach to organizing different segments of your application, making it simpler to manage and maintain. Whether working on a small-scale project or a larger application, integrating Django-Hosts can significantly elevate your routing strategy.