Understanding Unit Tests vs Integration Tests: A Comprehensive Guide
Written on
Chapter 1: The Importance of Testing in Software Development
In the realm of software development, the topic of testing often resurfaces. Although many articles delve into the intricacies of writing test code, few emphasize the fundamental purpose of these tests and their overarching role in the development process.
Testing is a vital component of software creation, regardless of the programming language employed. This article aims to reshape your perspective on code testing and clarify its significance.
Why Do We Test Code?
Every action we take serves a purpose, whether to bring joy, foster growth, or meet specific needs. The same principle applies to code testing. Reflect on your motivations:
- Are you testing because your supervisor mandates it?
- Is it due to a Git check that blocks PR merges if the tests fail?
- Are you merely following the crowd?
- Or do you genuinely enjoy the challenge of achieving 100% code coverage?
If any of your answers were "yes," reconsider your approach. The primary objective of writing tests should be to identify bugs and verify that your code functions as intended.
Let me reiterate:
THE GOAL IS TO CATCH BUGS AND CONFIRM YOUR CODE WORKS AS EXPECTED. (Apologies for the emphasis, but this is crucial to grasp.)
Common Justifications for Skipping Tests
I've encountered various excuses for neglecting tests, such as:
đź—Ł "We're in a rush; we can't afford the time for tests, as they're optional."
👉 It's crucial to recognize that neglecting test investment will inevitably result in compounded time lost during bug fixes and manual testing when new features are introduced. This often leads to increased stress, as uncertainty about your code amplifies, diverting attention from critical aspects like architecture and extensibility.
This situation reminds me of a quote by Einstein:
"Compound interest is the eighth wonder of the world. He who understands it earns it; he who doesn’t, pays it."
Consider compound interest as the extra time gained from implementing high-quality tests (which we’ll explore later).
Another frequent excuse is:
đź—Ł "We're under pressure to deliver features without tests; we can add them later."
👉 While this may be contextually valid, if urgency dictates a hasty delivery, it’s essential to commit to follow-ups and ensure that tests are written afterward. Neglecting this leaves gaps in your application that bugs can exploit.
Integration Tests vs Unit Tests
Confusion often arises regarding the distinctions between integration tests and unit tests. I, too, have struggled with this differentiation.
Does it truly matter what they are called?
The answer: it might, especially when communicating with colleagues about testing. However, the key consideration should always be the purpose of the tests: why you write them and how they assist you. (I urge you to keep this "test mantra" in mind as you write tests: always remember to question their purpose and utility.)
While integration and unit tests share similarities—both are crafted by developers to validate code functionality and swiftly identify bugs when new changes occur, both can be automated in pipelines, and both require setup and teardown processes—they also have distinct roles.
Integration Tests
These tests evaluate multiple components working in concert, designed to reveal issues arising from interactions between various code units.
Unit Tests
In contrast, unit tests focus on individual functions or methods, ensuring that each unit of code behaves correctly.
The challenge lies in the lack of clearly defined boundaries between these definitions, leading to confusion.
Let's consider a hypothetical e-commerce application featuring the following modules:
- Authentication Module: Responsible for sign-ups, log-ins, and log-outs.
- Product Module: Manages product catalog additions and detail retrieval (e.g., stock, price, description).
- Payment Module: Handles creating payments through an external service and interrogating payment statuses.
- Order Module: Facilitates adding products to draft orders, submitting orders, and retrieving order details.
Now, employing our "test mantra," we must ask:
What is the purpose of writing tests, and how can they help us?
Integration Tests Purpose
The aim of integration tests is to uncover issues in module connections. Although implementation specifics may not be fully defined, ensuring that inter-module contracts are respected is essential.
Begin by developing a list of use-cases you wish to test, such as:
- Setting up the testing environment (test database, cache adapters, mock servers).
- Calling the authentication module with sign-up parameters to create a user.
- Logging in with the parameters from the sign-up step.
- Retrieving a product list from the product module.
- Adding one product to the order via the order module.
- Submitting the order.
- Validating that the account creation and order registration were successful.
This method allows you to test multiple functionalities in a single end-to-end test while focusing on communication between modules.
Unit Tests Purpose
Conversely, unit tests should isolate functionalities to cover as many use-cases as possible. However, defining what constitutes a "unit" can be challenging:
- Is it a class method?
- A class?
- A module?
Many mistakenly perceive "units" as individual class methods, leading to inefficiencies. Instead, consider a "unit" as a feature of your module. For instance, fetching a product list could be treated as a single unit, where you test the service class’s output against expected results without delving into implementation specifics.
Thus, while unit tests and integration tests serve different purposes, they are not fundamentally dissimilar. You can often use similar testing tools across both types, with the primary difference being the focus on isolating features in unit tests.
I hope this clarification helps illuminate the distinctions between these types of tests. I would love to hear your thoughts and experiences on this topic—feel free to share your insights in the comments!
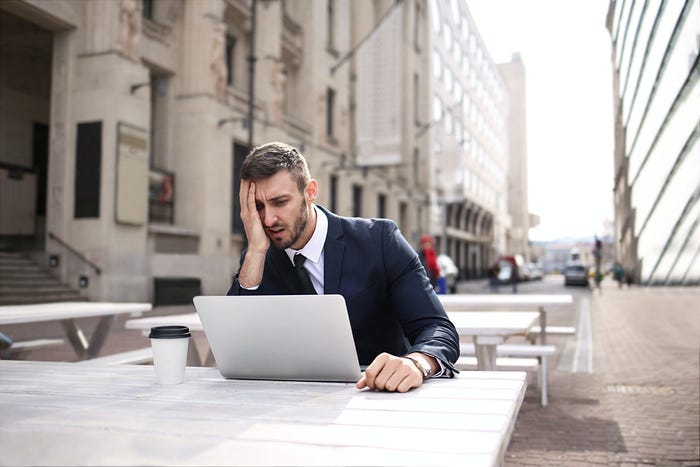