# Essential Python Operators to Master Early On
Written on
Chapter 1: Key Operators in Python
Understanding Python's operators is crucial for efficient coding. Here are ten operators you might regret not mastering sooner.
1. The Walrus Operator (:=)
The walrus operator serves a dual purpose. In the expression x := 5, it not only assigns the value 5 to the variable x, but it also evaluates to the value of x. This operator is useful for reducing the number of lines of code.
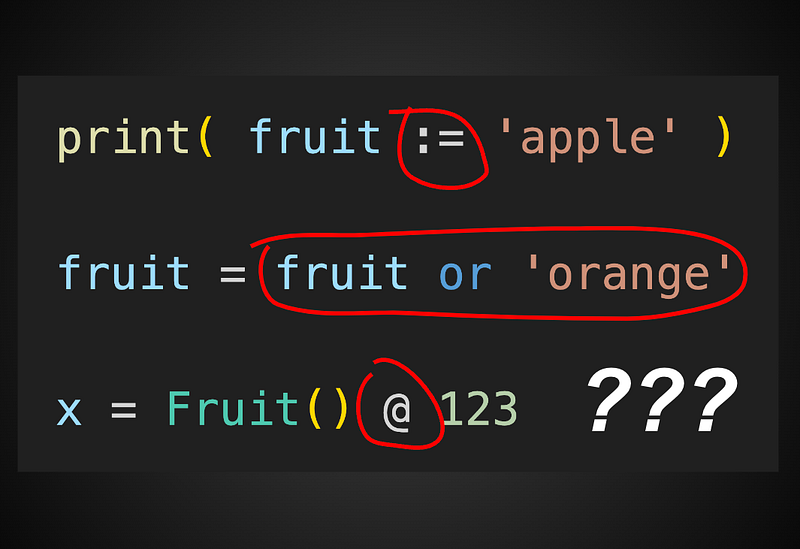
2. Using divmod()
When learning the basics of Python, many are introduced to the // operator for floor division and the % operator for remainders. However, the built-in divmod() function can accomplish both tasks simultaneously, returning a tuple of the quotient and the remainder.
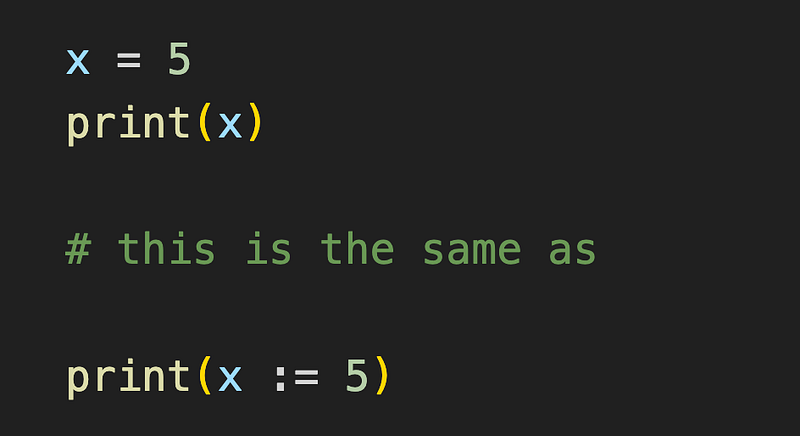
3. String Formatting with %
The % operator can be utilized for formatting strings, a method that has become somewhat outdated with the advent of string.format(). Nonetheless, this technique remains valid and is still present in some older Python codebases.
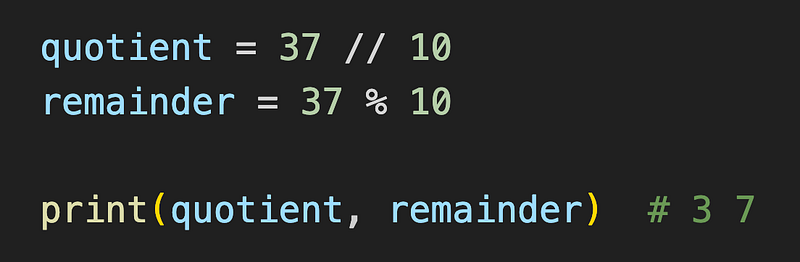
4. Matrix Multiplication with @
Surprisingly, Python includes a matrix multiplication operator represented by @. While basic data types like integers or floats cannot utilize this operator, types from the NumPy library can. Additionally, by defining the __matmul__ method in custom classes, you can tailor the behavior of the @ operator for your objects.
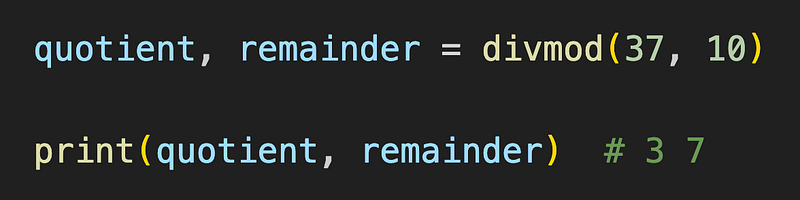
Quick Intermission
I recently authored a book titled 101 Things I Never Knew About Python, which compiles numerous tips and tricks that many developers might overlook. If you're eager to enhance your Python expertise, consider checking it out!
5. Magic Methods and Operators
When you use built-in operators in Python, you're essentially invoking their corresponding magic methods behind the scenes. For example:
- __add__ for +
- __sub__ for -
- __mul__ for *
- __truediv__ for /
- __floordiv__ for //
These magic methods can also be defined in custom classes, allowing for operator overloading on user-defined objects.
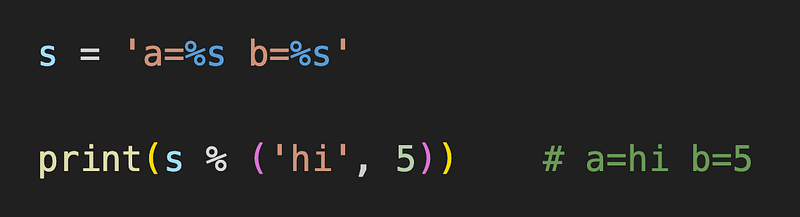
6. Compound Assignment Operators
If you have an integer variable x and want to increment it by 1, you can use compound assignment operators. For example, x += 1 is equivalent to x = x + 1. This applies to all arithmetic operators, simplifying your code.
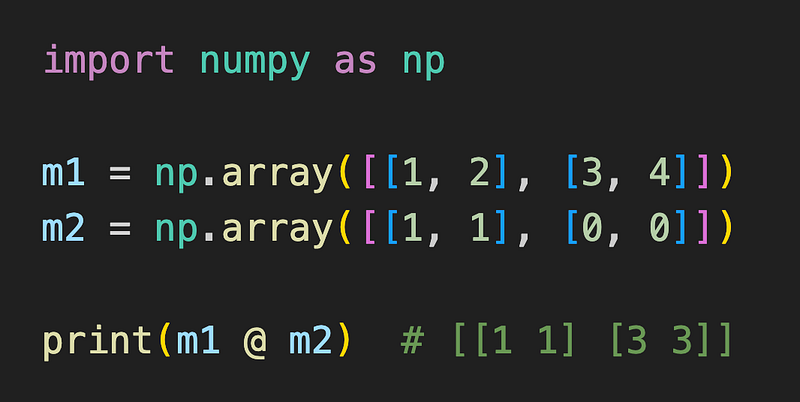
7. Type Hinting with ->
The -> operator is used for type hinting, indicating the expected return type of a function. For example, -> float denotes that the function is intended to return a float. While type hints provide guidance, they do not enforce strict type checking.
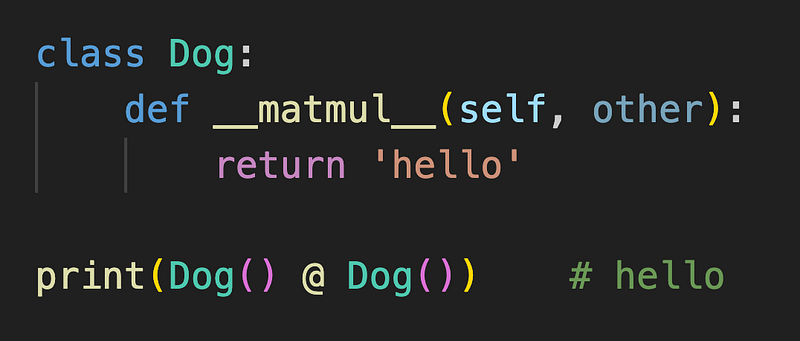
8. Utilizing the Ternary Operator
The ternary operator allows for compact conditional expressions. Instead of a lengthy if-else statement, you can use a single line to determine a variable's value.
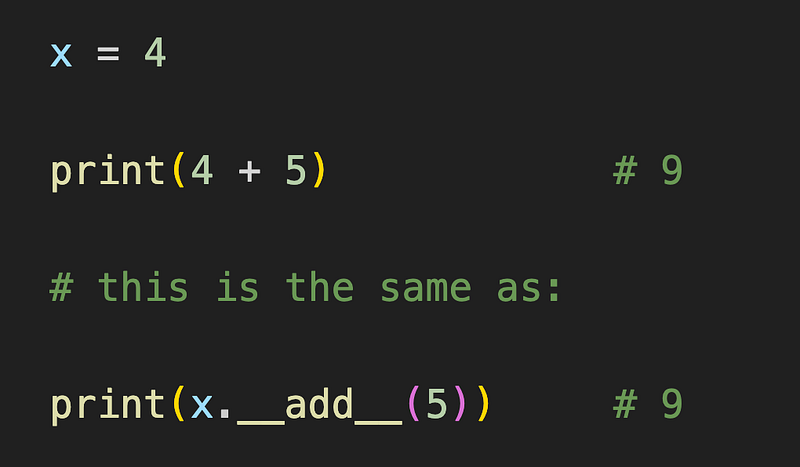
9. The 'None or Value' Expression
Using the expression None or 'apple', the output defaults to 'apple' due to the falsy nature of None. This technique is handy for ensuring that a variable is not None.
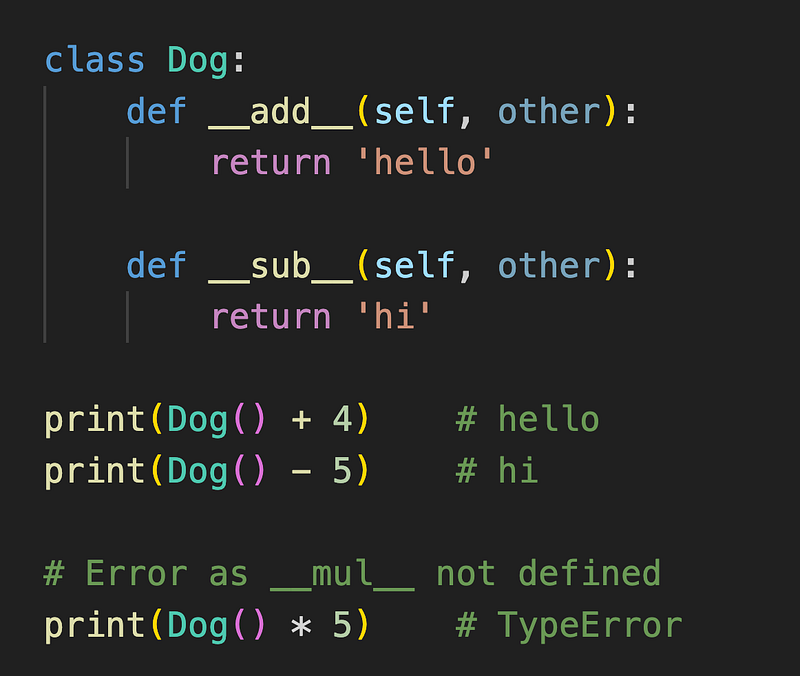
10. Understanding De Morgan’s Law
De Morgan’s Law is essential when using the not operator with conditions. For instance, not (A and B) translates to (not A or not B), which can be useful for logical expressions.
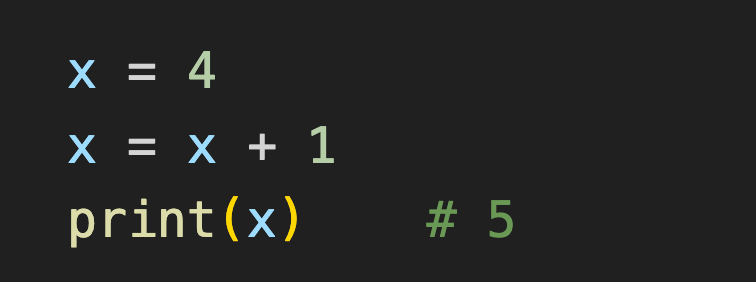
Chapter 2: Further Learning
To deepen your understanding of Python operators, consider watching these videos:
The first video titled "10 Nooby Mistakes Devs Often Make In Python" provides insights into common pitfalls in Python programming.
The second video, "Making a New Deep Learning Framework (ML in C Ep.02)," explores advanced topics in machine learning with practical applications.